BALANCED SEARCH TREES
2-3 trees. Understand how to search and insert.
Understand why insertion technique leads to perfect balance and symmetric order.
Terminology. Symmetric order. Perfect balance.
Performance of 2-3 trees. Tree height is between log_3 N and log_2 N. All paths are of the same height.
1-1 correspondence between LLRBs and 2-3 trees. Understand how to map a 2-3 tree to an LLRB and vice versa.
LLRBs. BST such that no node has two red links touching it; perfect black balance; red links lean left.
LLRB get. Exactly the same as regular BST get.
Storing color. A node's color is the same as the edge connecting that node to its parent.
LLRB insert. Insert as usual, then apply rotations recursively as follows, working upwards from the new node.
- Case 3: A parent node has a black left child and a red right child, so rotate the parent left.
The former right child is now the boss. Reminder: null links are considered black for the purposes of deciding cases.
- Case 2: A grandparent node has a red left child whose left child is also red.
Rotate this grandparent right (so that one in the middle is now the boss).
- Case 1: A parent node has two red children. Flip colors.
Conveniently, we can always apply case 3, case 2, then case 1 to every node in a tree,
and we can guarantee that the entire tree will be an LLRB.
LLRB performance. Perfect black balance ensures worst case performance for get and insert is ~ 2 log_2 N.
Recommended Problems
C level
- Given an LLRB, is there exactly one corresponding 2-3 tree? Given a 2-3 tree, is the exactly one corresponding LLRB?
- Draw a 2-3 tree with all 3 nodes. Why is the height log3(N)?
- How many compares does it take in the worst case to decide whether to take the left, middle, or right link from a 3 node?
- Spring 2012 Midterm, #5.
-
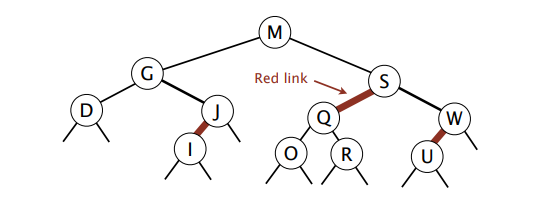
(a) Circle the keys whose insertion will cause either rotations and/or color flips.
A B C E F H K L N P T V X Y Z
(b) Draw and give the level order traversal of the tree that results after inserting the
key Z. Write the level order traversal. Clearly indicate red nodes.
(c) In plain English, describe the function of mystery().
public boolean mystery() {
return mystery(root, null, null);
}
private boolean mystery(Node x, Key a, Key b) {
if (x == null) return true;
if (a != null && x.key.compareTo(a) <= 0) return false;
if (b != null && x.key.compareTo(b) >= 0) return false;
return mystery(x.left, a, x.key) && mystery(x.right, x.key, b);
}
Answers
(a) E F H K L and P T V X Y Z.
(b) S M W G Q U Z D J O R I. The red nodes are M and I.
(c) Returns true if the Node is the root of a properly constructed BST.
Best approach is probably just to draw some arbitrary BST with
integer keys and trace the algorithm, relying on human inductive
skill to arrive at the answer.
-
(a) Label each node in the following binary tree with numbers from the set {2,26,10,27,20,15,42} so that
it is a legal Binary Search Tree. (Hint: use the back of the page as scratch space, and only write down the
answer once you have it.)
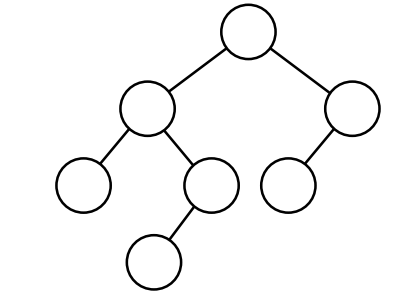
(b) Now label each edge in the figure with r or b, denoting RED and BLACK, so that the tree is a legal
Left-Leaning Red-Black Tree
Answers
B level
- Continuing the previous question.
(c) Considering your labeling in (b), is it possible to assign different red/black labels and still satisfy the
LLRB-tree conditions? (Answer yes if a different labeling is possible, or no if your labeling in (b) is unique.)
(d) If the answer to (c) is yes, draw and label the second tree. If the answer is no, how do you know that the red-black labeled tree must be unique?
Answers
(c) no
(d) Sample answer:
Thinking about the equivalence to a 2-3 tree, 27-42 must be a 3-node since
42 can't have only 1 child. Therefore 42-27 is red. Therefore every path
from root to leaf has to have 1 black edge. Since red edges can't be
consecutive, 10-20 must be black and 26-10 and 20-15 red. Finally, 10-2
must be black because 26-10 was red.
- For a 2-3 tree of height h, what is the best case number of compares to find a key? The worst case?
A level
- Show that in the worst case, we need to perform order log N LLRB operations
(rotation and color flipping) after an insert (not as hard as it might seem).
Give an example of a tree which requires log N LLRB operations after a single insert (also not so hard).